C# application connecting to Facebook
Overview
This is a simple example of how a C# console app (.NET Framework) can:
- Connect to the Facebook REST API
- Download ‘leads’ information from a Facebook leads form
(The class used to achieve this being the HttpClient class.)
Steps
1.) Log onto Facebook and record the leads form Id (assuming that its already been created).
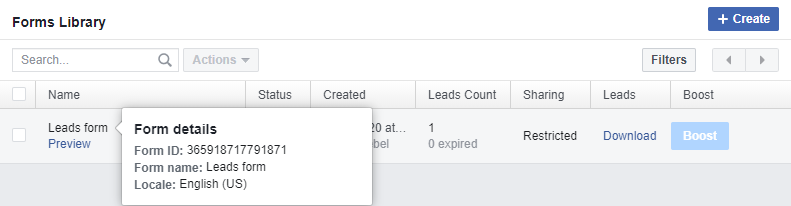
2.) Register to use developers.facebook.com/apps/ and create a Facebook app by selecting ‘Manage Business Integrations’.
3.) Use the Facebook Graph API explorer to generate an access token and use this token to validate that the API is working as expected.
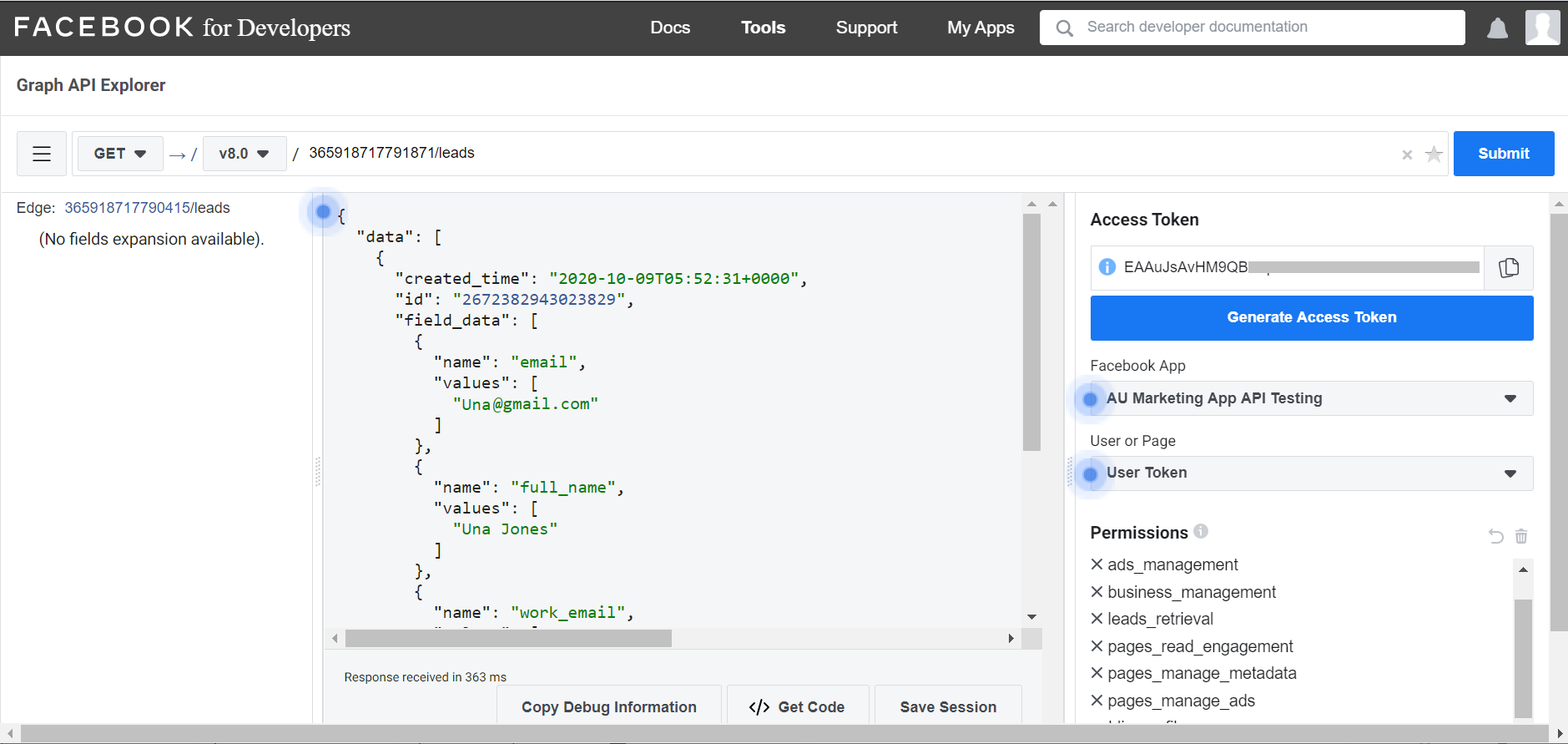
4.) Write the C# code displayed in Figure 3
Please note the following about the C# code:
On line 2 (Figure 3), an asynchronous call is made to Facebook. This is a non blocking call and will suspend RetrieveLeadsfromFacebookForm() and return immediately to the caller (line 1). The app only completes when the async task resumes and completes processing and the FacebookLeads object on line 1 is populated.
On line 3, the call ‘facebookLeads = JsonConvert.DeserializeObject…’ is to deserialise the Facebook JSON response into an object that can be accessed from within C#.
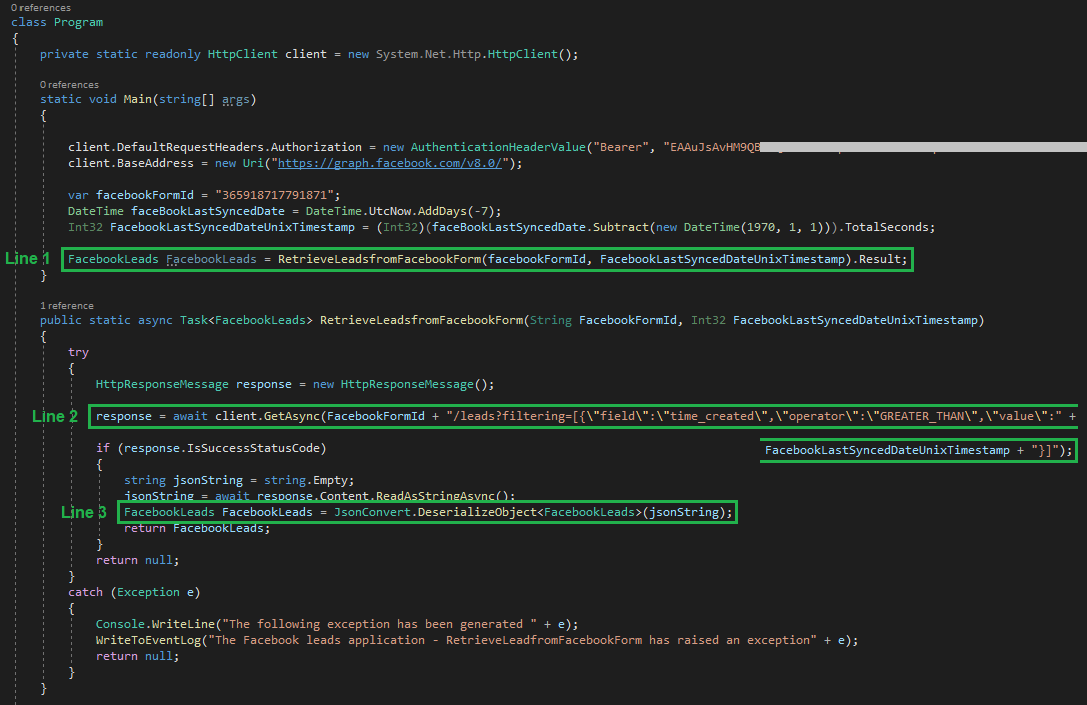
The Facebook JSON response deserialisation is based on the following schema. (This schema was generated in Visual Studio by pasting the Facebook JSON response by selecting ‘Paste Special’, ‘Paste JSON As Classes’.)
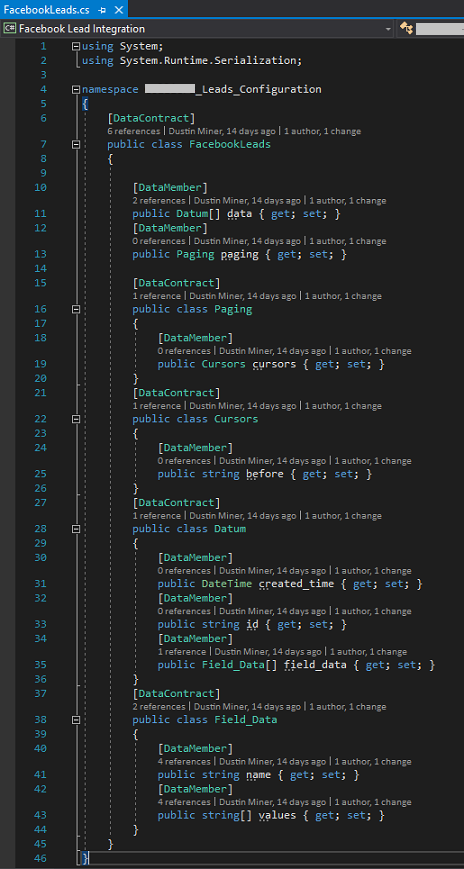
The format of the generated facebookLeads object is shown in Figure 5 below.
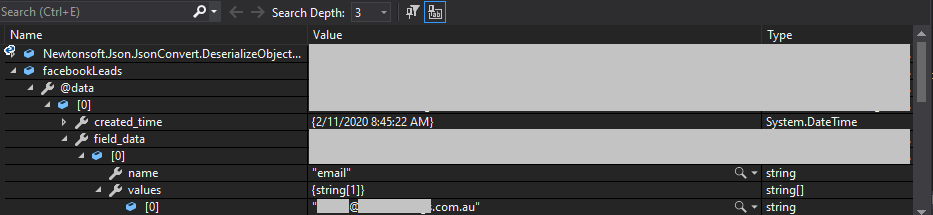
Figure 6 illustrates how the values are extracted from the JSON response.
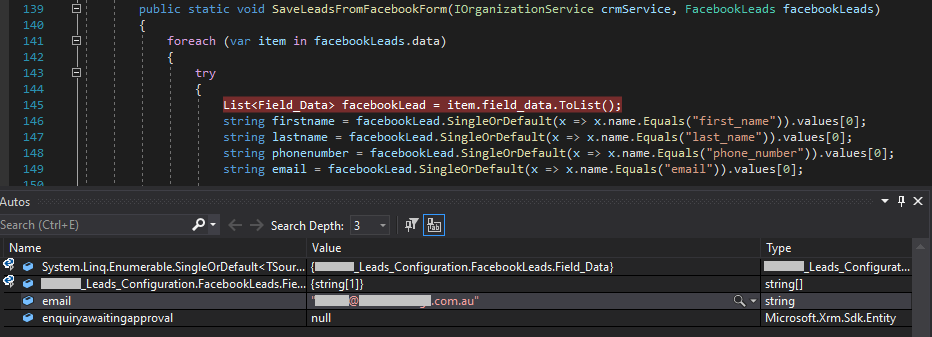
Referring to line 146 in Figure 6, the first ‘x’ represents a list of name/value pairs. The value returned is the value associated to the name ‘first_name’. Considering this value could be null, the statement should be updated to …name.Equals(“first_name”))?.values[0]. This would mean that values[0] would only be executed if “first_name” exists. The ‘question mark/full stop’ is called a null-conditional/Elvis operator.
References
https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/operators/member-access-operators