Scenario
Contoso Water Purifiers uses a Facebook page to advertise their products and generate leads. When a potential customer views the page and shows interest in a particular product, they enter their contact details into leads form (which is available on the Facebook page). To facilitate easy retrieval of these leads, the business tasks their IT department with writing an app that downloads customer leads from Facebook using the Facebook REST API. Although not discussed in this post, it is expected that these leads will be entered into the business’s internal IT systems.
Implementation
This section demonstrates how a developer from the IT department implements this requirement. The steps outline how to write a C# console app (.NET Framework) to connect to the Facebook REST API and download leads.
1.) Retrieve the leads form Id from within the business’ Facebook page (Figure 1)
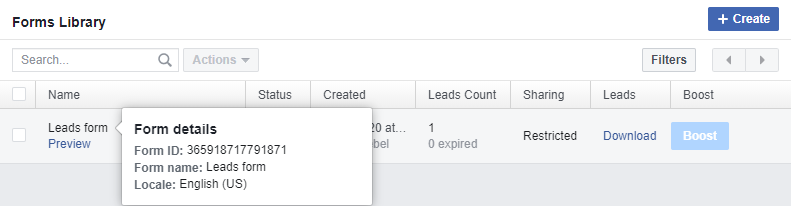
2.) Register to use developers.facebook.com/apps/ and create a Facebook app by selecting ‘Manage Business Integrations’.
3.) Use the Facebook Graph API explorer to generate an access token and use this token to validate that the Facebook REST API is working as expected (Figure 2).
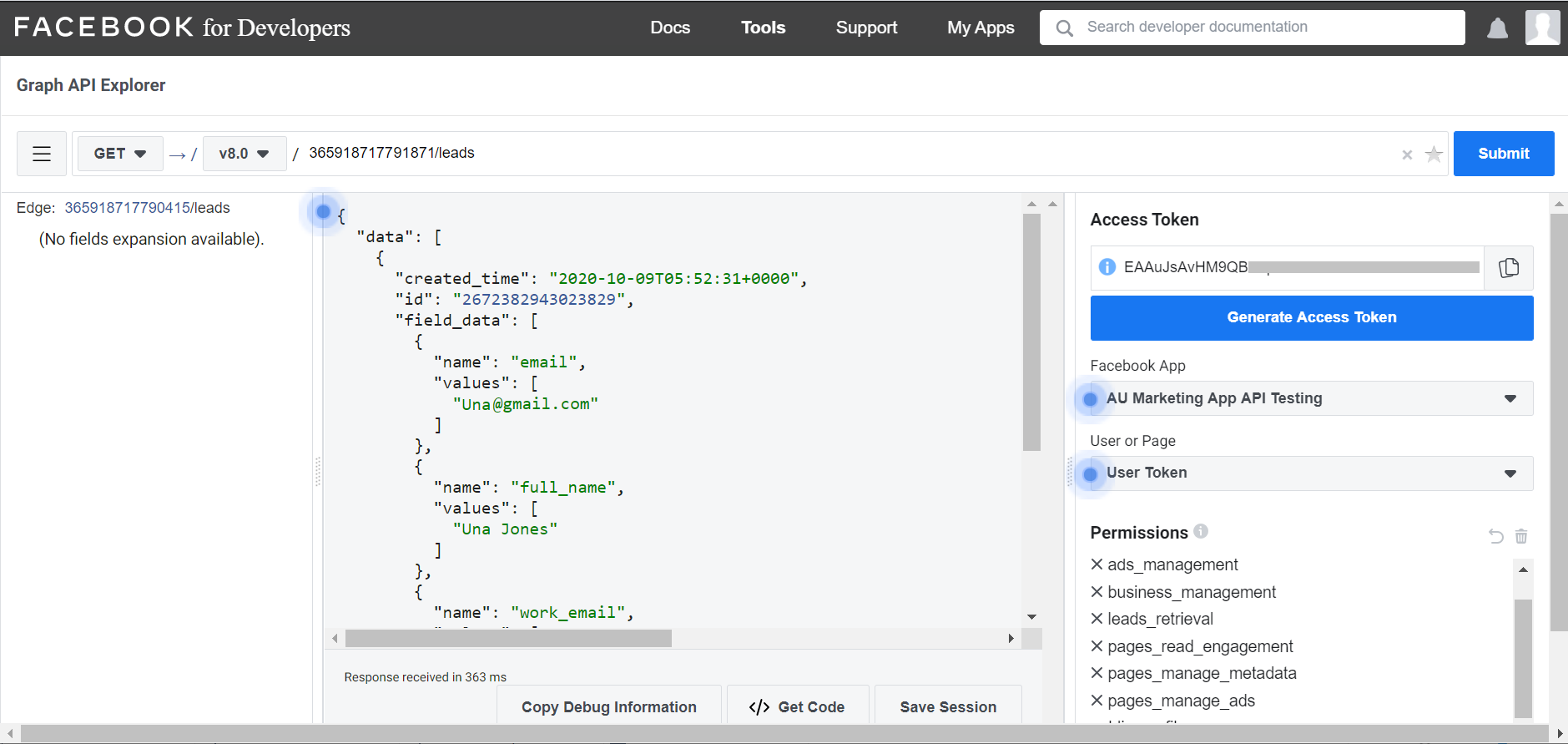
4.) Take the Facebook REST API JSON response and paste it into Visual Studio by selecting ‘Paste Special’, ‘Paste JSON As Classes’. This generates the schema ‘model’ shown in Figure 3. The Facebook JSON response deserialisation is based on this schema (Figure 4)
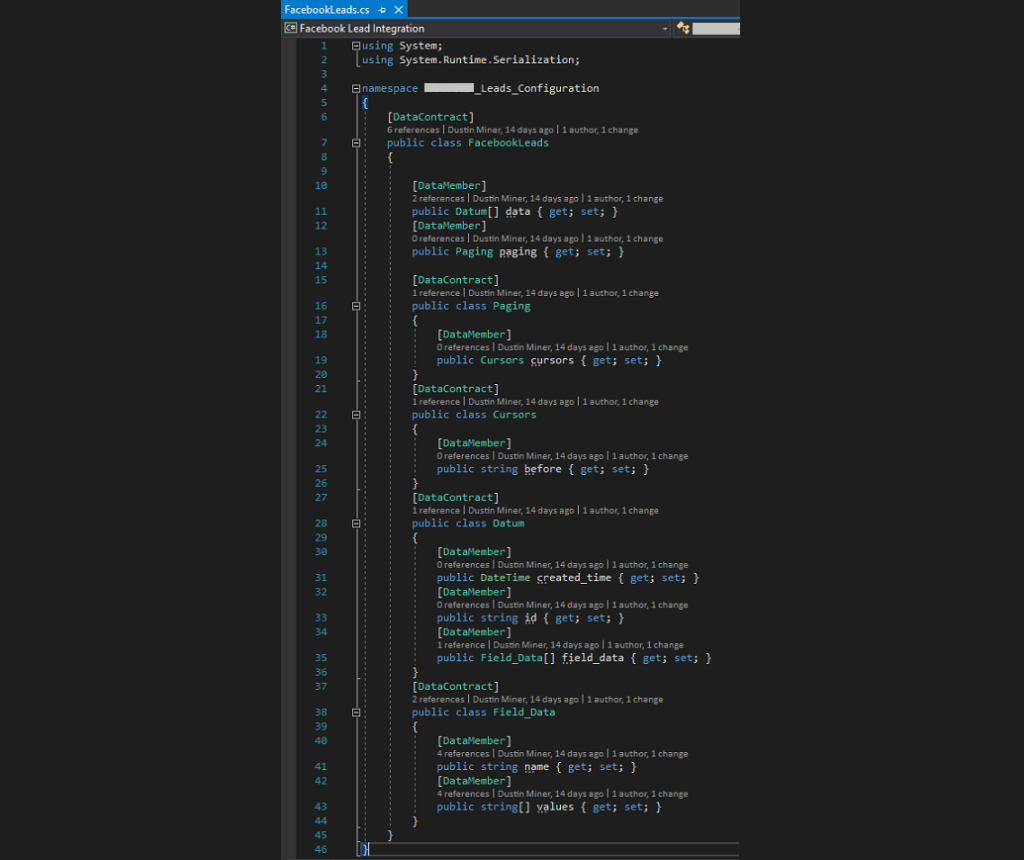
5.) Write the C# code displayed in Figure 4
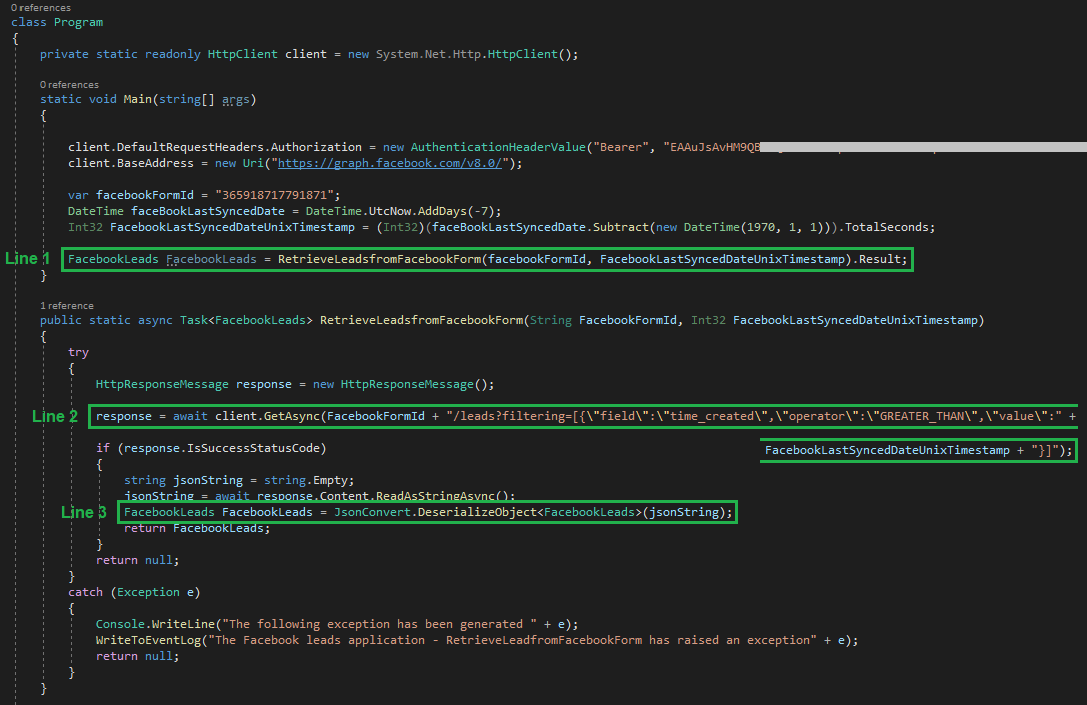
6.) Run the C# console app. Referring to Figure 4
- The HttpClient GetAsync() call (line 2) executes and makes an asynchronous call to the Facebook REST API. This is a non blocking call and will suspend the function RetrieveLeadsfromFacebookForm() and return immediately to the caller (line 1).
- The async call returns, and line 3 executes. This deseralises the Facebook REST API JSON response into an object called FacebookLeads
- The FacebookLeads object on line 1 is populated (Figure 5).
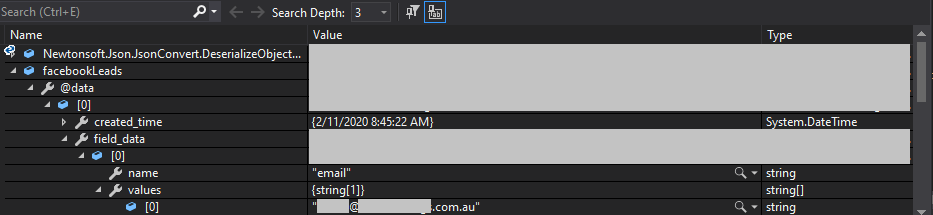
7.) View the values extracted from the JSON response in Figure 6
Referring to line 146 in Figure 6, the first ‘x’ represents a list of name/value pairs. The value returned is the value associated to the name ‘first_name’.
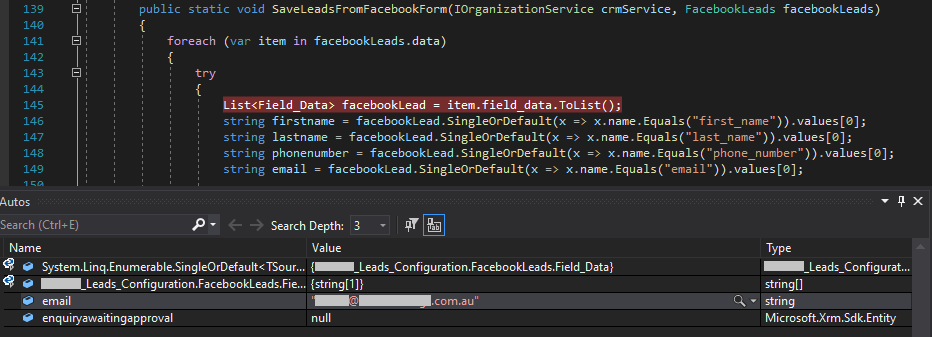
Further Reading
Download leads from the Facebook API issue
References
https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/operators/member-access-operators
c#6 – Null-Conditional Operator
https://learn.microsoft.com/en-us/dotnet/api/system.net.http.httpclient?view=net-8.0